AWS Infrastructure part1 - Lambda, SQS and more
Desinging infrastructure using AWS services
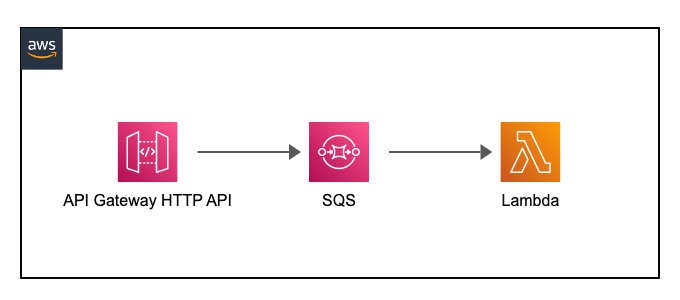
AWS has hundreds of different products and Simple Queue Service (SQS)
is one of them (one of the earliest ones that became available). I wrote unit tests for SQS based services but I figured that I did not know how they operate exactly, nor I knew the exact reason for using them. Having created test AWS account, I thought it would be great to make some examples and understand the mechanism underneath it. On top of SQS, I am also going to explore AWS Lambda
and API Gateway
to build a sample application.
Understanding SQS
We first need to understand why the hack we need SQS. I built couple test applications and they seem to work just fine?
Why do we need SQS?
Applications nowadays are collection of distributed systems, and to efficiently pass data or logic b/w these services, you need Distributed Message Broker Service which helps establish reliable, secure, and decoupled communication. One of the best features of SQS is that it lets you transmit any volume of data, at any level of throughput, which gets dynamically created and scale automatically. SQS always ensures that your message is delivered at least once, and also allows multiple consumers and senders to communicate using the same Message Queue. By default it offers:
Standard Queues
: give the highest throughput, best-effort ordering, and at least one delivery.FIFO Queues
: First-In, First-Out Queues ensure that messages are processed only once, in the sequence in which they are sent.
A queue is just a temporary repository for messages awaiting processing and acts as a buffer between the component producer and the consumer. SQS provides an HTTP API over which applications can submit items into and read items out of a queue.
Use cases of SQS Service
Okay, now we know what understand what SQS is very roughly. In what kind of circumstances would you use it?
For example, let’s say you have a service where people upload photos from their mobile devices. Once the photos are uploaded your service needs to do a bunch of processing of the photos, e.g. scaling them to different sizes, applying different filters, extracting metadata. Each user’s request will be put into a queue asynchronously, waiting to be processed. Once it’s processed, completed messages are sent back. When the server dies for some reason (Services like Kubernetes will restore them), the messages will be go back to the queue and will be picked up by another server. There are things like Visibility timeout
in which when the message is not deleted by the message consumer in time, the message will return to the queue. During this period, no other consumers can receive or process the message.
So reiterate, SQS does not do any load-balancing or actual processing of the requests. It just acts as a communication bridge between applications so that status of jobs can be monitored and requests are properly fulfilled without being lost.
Understanding Lambda and API Gateway
Next up is AWS Lambda and API Gateway.
Serverless cloud computing models automatically scales up your application so you no longer need to have infrastructure-related concerns about surge in requests. Within the serverless architecture, there are something called Backend as a Service (BaaS)
and Function as a Service (FaaS)
models.
BaaS
hosts and replaces a single component as a whole (e.g Firebase authentication service, AWS Amplify).FaaS
is a type of service in which all features of application are deployed into individual single feature and then each feature is individually hosted by the provider (e.g AWS Lambda)
It’s not like one approach is better than the other. It’s just two different approaches for serverless architecture. AWS Lambda
is a typical example of FaaS based architecture, as we have bare minimal level of a function that gets invoked based on certain predetermined events, and gets torn down as soon as they are done being processed.
How does Lambda work under the hood? A Lambda function runs inside a microVM (micro virtual machine). When an invocation is received, Lambda will launch a new microVM and load the code package in memory to serve the request. The time taken by this process is called startup time. MicroVMs are a new way of looking at virtual machines. Rather than being general purpose and providing all the potential functionality a guest operating system may require, microVMs seek to address the problems of performance and resource efficiency by specializing for specific use cases. By implementing a minimal set of features and emulated devices, microVM hypervisors can be extremely fast with low overhead. Boot times can be measured in milliseconds (as opposed to minutes for traditional virtual machines).
How does AWS Lambda connect with API Gatway?
AWS Lambda’s functions are event driven, and API Gateway
exposes REST API endpoint online which acts as a trigger to scale AWS Lambda function up and down.
2.1 - Simple example with Lambda and API Gateway
We now know have all the preliminary knowledge to code something up. Let’s go to AWS console and make a Lambda function and corresponding API Gateway hook.